Twinkling Stars using Java Applet
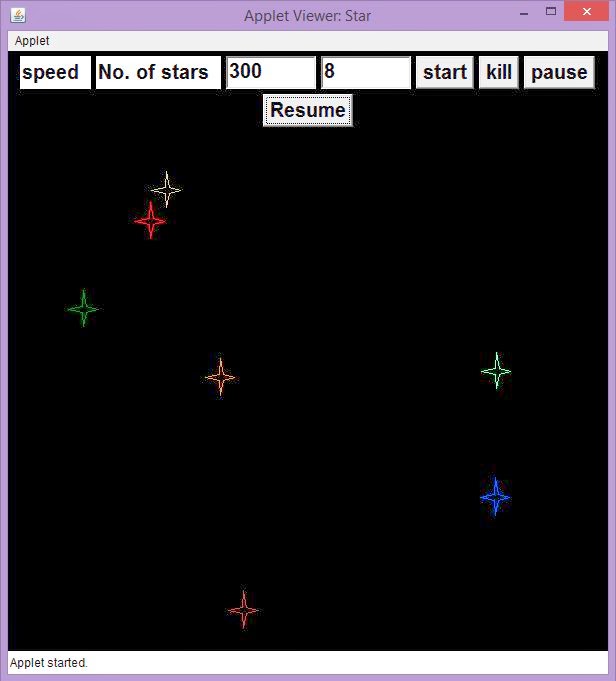
import java.applet.Applet;
import java.awt.*;
import java.awt.event.*;
/*<applet code="Star" width=600 height=600></applet>*/
public class Star extends Applet implements ActionListener,Runnable
{
Thread t;
Button b1,b2,b3,b4;
TextField tf1,tf2;
Label l1,l2;
Font f;
public void init()
{
setBackground(Color.black);
l1= new Label("speed");
l2 =new Label("No. of stars");
f=new Font("Arial",Font.BOLD,20);
l1.setFont(f);
l2.setFont(f);
l1.setBackground(Color.white);
l2.setBackground(Color.white);
tf1= new TextField(6);
tf1.setFont(f);
tf2 = new TextField(6);
tf2.setFont(f);
b1 = new Button("start");
b2 = new Button("kill");
b3 = new Button("pause");
b4 = new Button("Resume");
b1.setFont(f);
b2.setFont(f);
b3.setFont(f);
b4.setFont(f);
b1.addActionListener(this);
b2.addActionListener(this);
b3.addActionListener(this);
b4.addActionListener(this);
add(l1);
add(l2);
add(tf1);
add(tf2);
add(b1);
add(b2);
add(b3);
add(b4);
}
public void actionPerformed(ActionEvent ae)
{
if((ae.getSource()==b1)&&(t==null))
{
t = new Thread(this);
t.start();
}
else if((ae.getSource()==b2)&&(t!=null))
{
t.stop();
t=null;
}
else if((ae.getSource()==b3)&&(t!=null))
{
t.suspend();
}
else if((ae.getSource()==b4)&&(t!=null))
{
t.resume();
}
}
public void run()
{
while(true)
{
repaint();
try{
Thread.sleep(Long.parseLong(tf1.getText()));
}catch(InterruptedException ie)
{
}
}
}
public void paint(Graphics g)
{
for(int i=0;i<Integer.parseInt(tf2.getText());i++)
{
g.setColor(new Color(((int)(Math.random()*1000))%254,((int)(Math.random()*1000))%254,((int)(Math.random()*1000))%254));
star(g,((int)(Math.random()*1000))%600,((int)(Math.random()*1000))%600);
}
}
static void star(Graphics g,int x,int y){
Point[] points = new Point[9];
points[0] = new Point(28+x, 5+y);
points[1] = new Point(27+x, 21+y);
points[2] = new Point(13+x, 24+y);
points[3] = new Point(27+x, 27+y);
points[4] = new Point(28+x, 41+y);
points[5] = new Point(31+x, 27+y);
points[6] = new Point(42+x, 24+y);
points[7] = new Point(31+x, 21+y);
points[8] = new Point(28+x, 5+y);
int i;
for (i=1; i<points.length; i++)
g.drawLine(points[i-1].x, points[i-1].y, points[i].x, points[i].y);
}
}
3 comments:
Not Working Dude!!
This code works fittingly ... You would have mistyped anything ... Anyway if problem persists meet me personally or comment your errors here !
How do I run this code dude??
Post a Comment